반응형
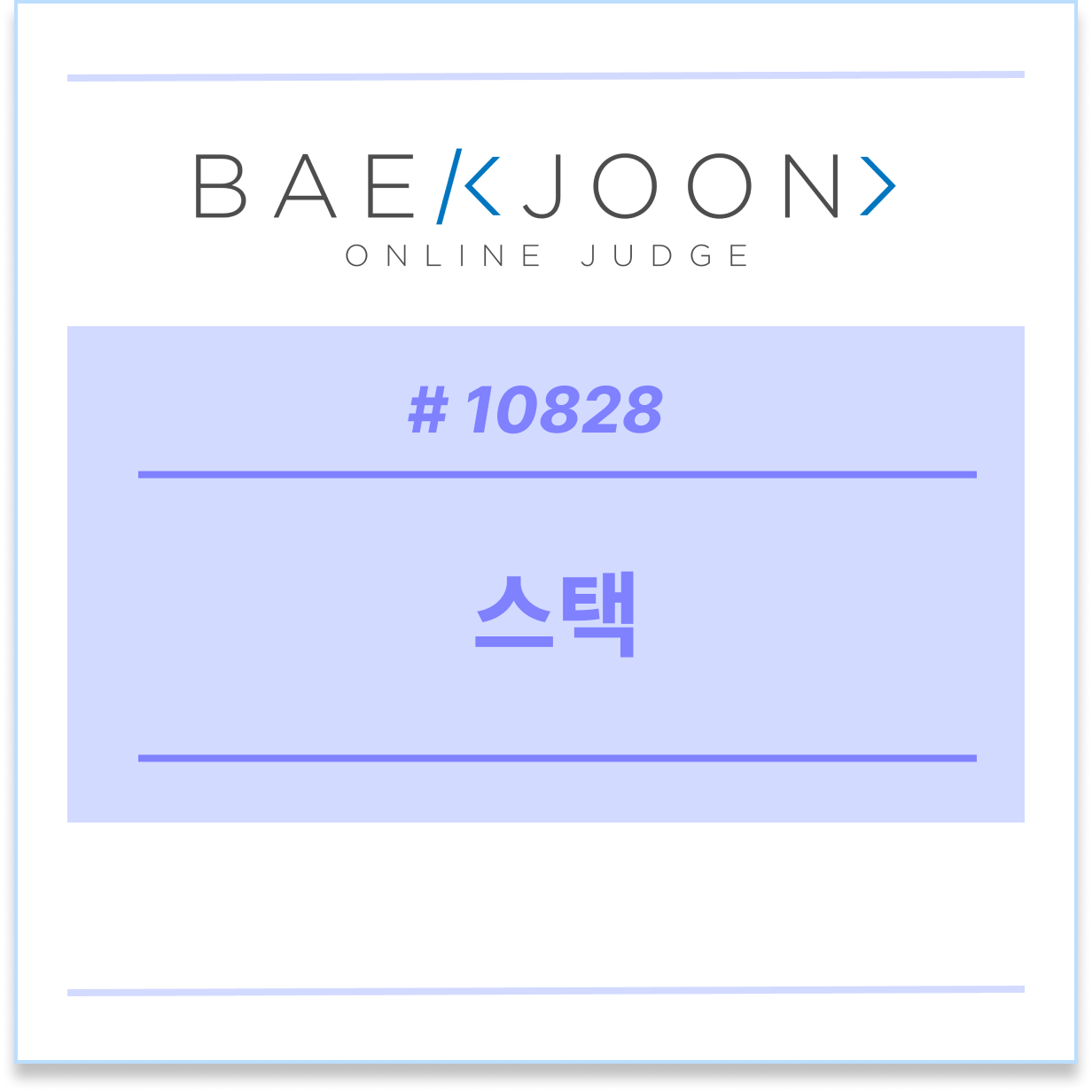
문제
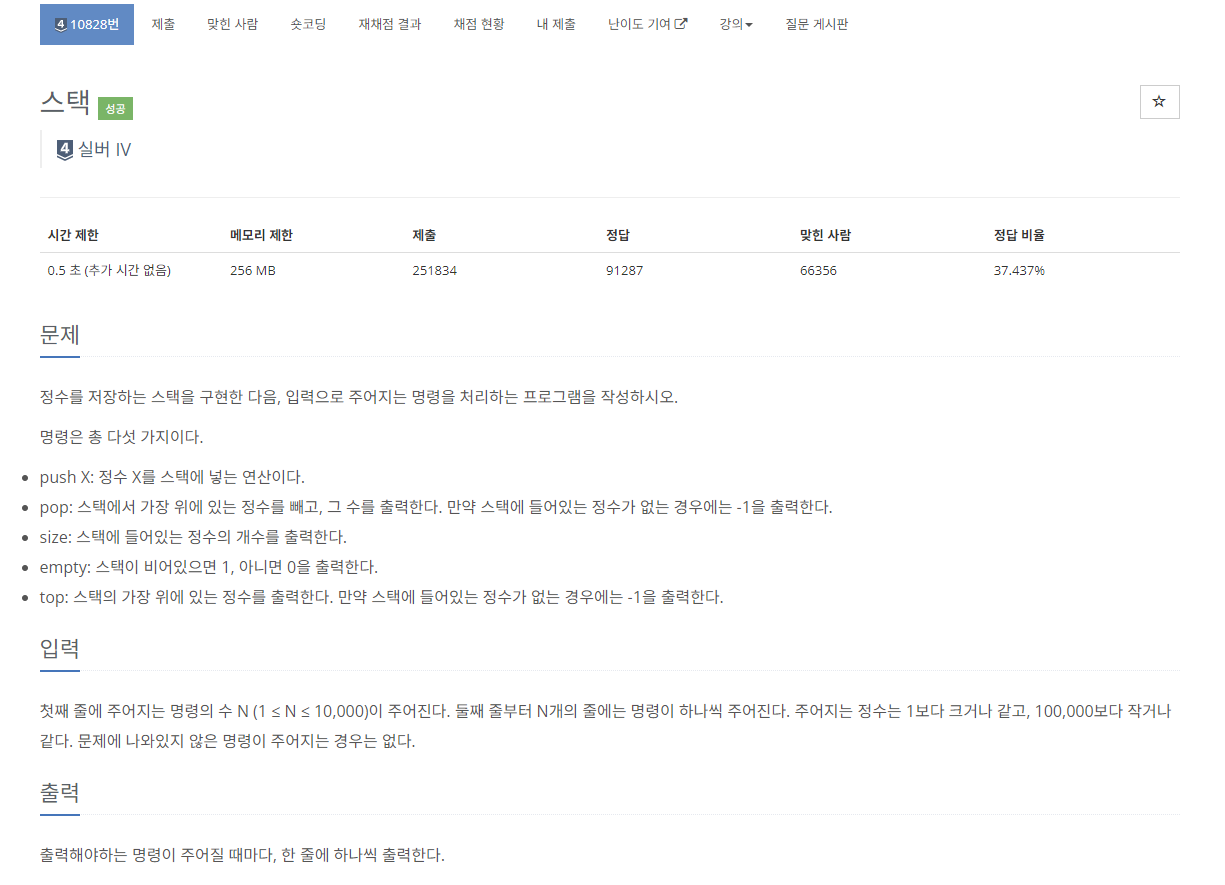
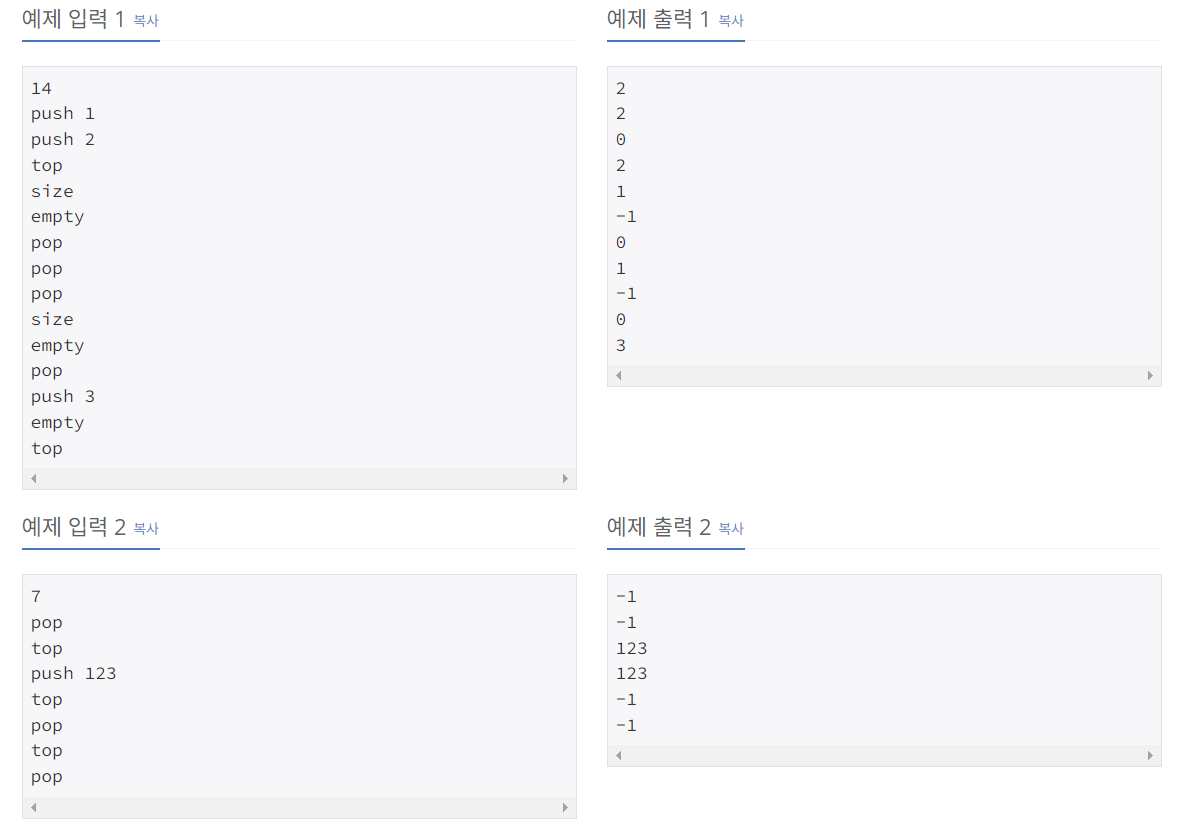
풀이
스택(Stack)은 LIFO(Last In First Out, 후입선출) 구조이다.
스택은 컨테이너의 한 쪽 끝에서만 데이터를 삽입하거나 삭제할 수 있다.
따라서, 한쪽 끝이 아닌 위치에 있는 데이터는 접근하거나 변경할 수 없다.
스택을 구현하기 위해 std::deque 와 std::stack을 사용할 수 있고, 풀이에 후자를 사용했다.
// std::deque를 사용해 stack 구현
#include <deque>
std::deque<int> stk1;
stk1.push_back(1);
stk1.push_back(2);
stk1.pop_back();
// std::stack를 사용해 stack 구현
#include <stack>
std::stack<int> stk2;
stk2.push(1);
stk2.push(2);
stk2.pop();
코드
#include <iostream>
#include <string>
#include <stack>
using namespace std;
int main() {
int N, num;
cin >> N;
stack<int> st;
string str;
for (int i = 0; i < N; i++) {
cin >> str;
if (str == "push") {
cin >> num;
st.push(num);
}
else if (str == "pop") {
if (st.empty()) {
cout << "-1" << endl;
}
else {
cout << st.top() << endl;
st.pop();
}
}
else if (str == "size") {
cout << st.size() << endl;
}
else if (str == "empty") {
if (st.empty()) {
cout << "1" << endl;
}
else {
cout << "0" << endl;
}
}
else if (str == "top") {
if (st.empty()) {
cout << "-1" << endl;
}
else {
cout << st.top() << endl;
}
}
}
return 0;
}
결과
반응형
'Programming > C++ - 백준' 카테고리의 다른 글
[백준] 11866번 : 요세푸스 문제 0 (C++) (0) | 2024.03.13 |
---|---|
[백준] 10845번 : 큐 (C++) (0) | 2024.03.11 |
[백준] 2798번 : 블랙잭 (C++) (0) | 2024.03.10 |
[백준] 2869번 : 달팽이는 올라가고 싶다 (C++) (0) | 2024.03.07 |
[백준] 5597번 : 과제 안 내신 분..? (C++) (0) | 2024.03.05 |
댓글