반응형
문제
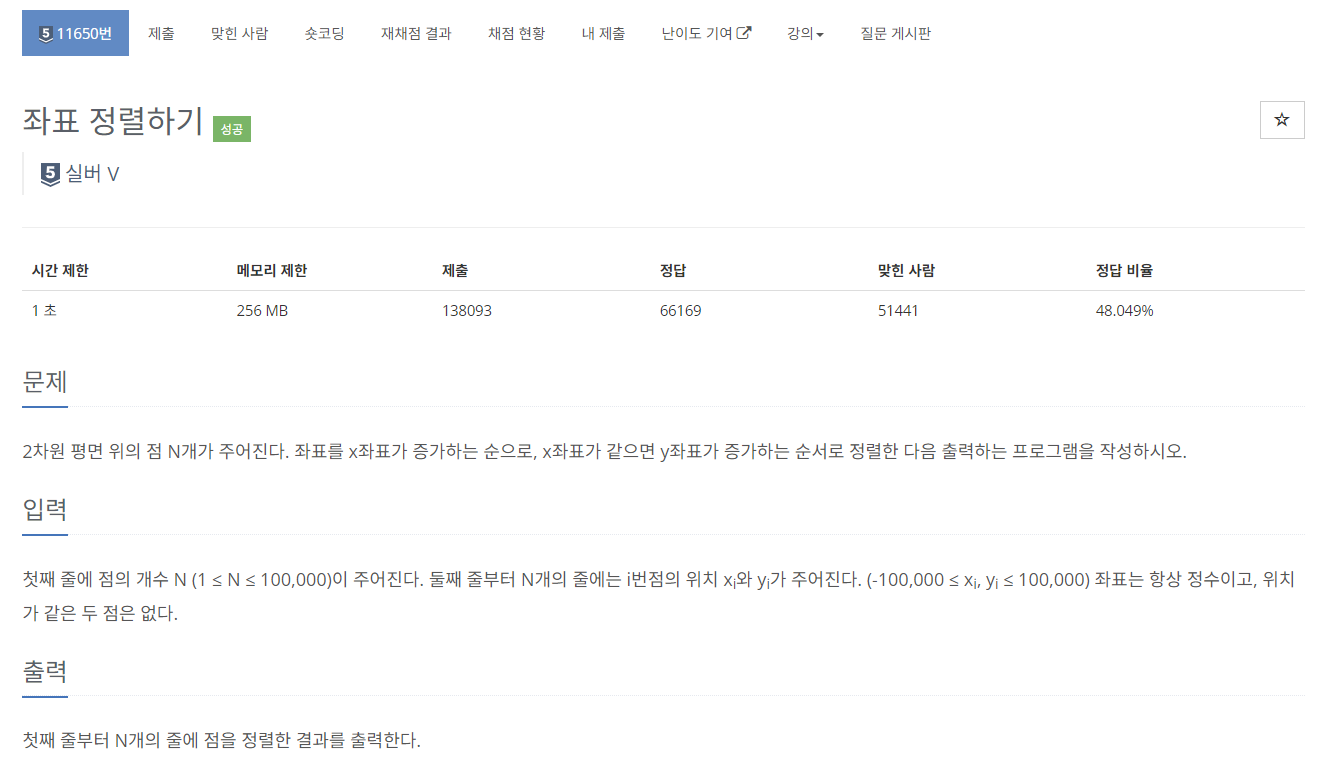
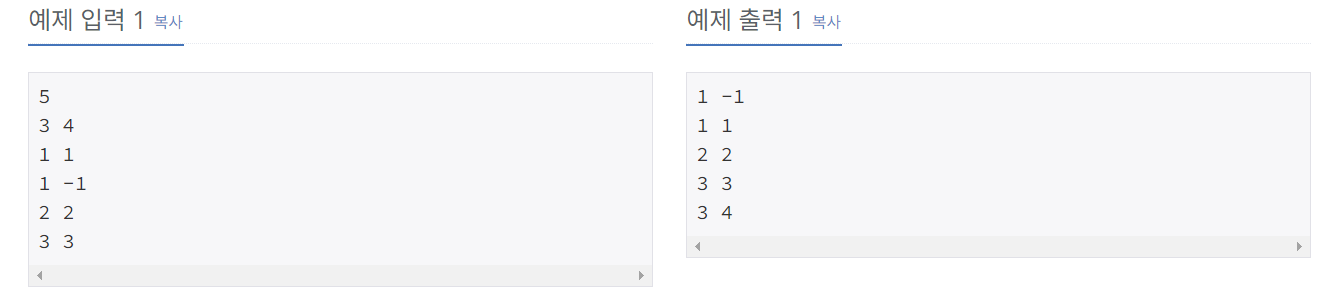
풀이
'이차원 벡터'와 '구조체를 이용한 배열'로 풀이했다. 총 세 가지의 풀이로 문제를 해결했다!
[ '이차원 벡터' 풀이 ]
1. 이차원 벡터 선언
vector<pair<int, int>> v; // 이차원 벡터 선언
2. 이차원 벡터 요소 입력
for (int i = 0; i < n; i++){
cin >> x >> y;
// 첫 번째 - 이차원 벡터 입력 방법
v.push_back({x, y});
// 두 번째 - 이차원 벡터 입력 방법
v.push_back(make_pair(x, y));
}
3. 오름차순 정렬
// 사용자 지정 compare 생략 - 오름차순 정렬이기 때문에 가능
sort(v.begin(), v.end()); // 오름차순
sort(v.begin(), v.end(), less<int>()); // 오름차순
// 사용자 지정 compare
bool compare(pair<int, int>a, pair<int, int>b) {
if (a.first == b.first) { // x가 같은 경우에만,
return a.second < b.second; // y를 기준으로 오름차순
}
else {
return a.first < b.first; // x를 기준으로 오름차순
}
}
sort(v.begin(), v.end(), comapare);
4. 이차원 배열 출력
for (int i = 0; i < n; i++) {
cout << v[i].first << " "<< v[i].second << "\n";
}
[ '구조체를 이용한 배열' 풀이 ]
1. array를 만들기 위한 구조체(struct) 생성
struct coordinate { // array 구조체 생성
int x, y;
};
2. 구조체 array 선언
struct coordinate arr[100001];
처음에는, int main() 안에 array를 선언했으나 아래와 같은 '함수가 스택의 바이트를 사용합니다. 일부 데이터를 힙으로 이동하는 것이 좋습니다'라는 경고가 발생했다. 이는 전역변수로 바꾸어 해결했다.
3. 값 입력받기
for (int i = 0; i < n; i++) {
cin >> arr[i].x >> arr[i].y;
}
4. 오름차순 정렬
// 사용자 지정 compare
bool compare(coordinate a, coordinate b) {
if (a.x == b.x) {
return a.y < b.y;
}
else {
return a.x < b.x;
}
}
sort(arr, arr+ n, compare);
5. 배열 출력
for (int i = 0; i < n; i++) {
cout << arr[i].x << " " << arr[i].y << "\n";
}
첫 번째 코드 - Vector & 사용자 지정 Compare (X)
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
int main(){
int n, x, y;
vector<pair<int, int>> v; // 이차원 벡터 선언
cin >> n;
for (int i = 0; i < n; i++){
cin >> x >> y;
// 첫 번째 - 이차원 벡터 입력 방법
v.push_back({x, y});
// 두 번째 - 이차원 벡터 입력 방법
// v.push_back(make_pair(x, y));
}
sort(v.begin(), v.end()); // 오름차순
// sort(v.begin(), v.end(), less<int>()); // 오름차순
for (int i = 0; i < n; i++) {
cout << v[i].first << " "<< v[i].second << "\n";
}
return 0;
}
두 번째 코드 - Vector & 사용자 지정 Compare (O)
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
bool compare(pair<int, int>a, pair<int, int>b) {
if (a.first == b.first) { // x가 같은 경우에만,
return a.second < b.second; // y를 기준으로 오름차순
}
else {
return a.first < b.first; // x를 기준으로 오름차순
}
}
int main(){
int n, x, y;
vector<pair<int, int>> v; // 이차원 벡터 선언
cin >> n;
for (int i = 0; i < n; i++){
cin >> x >> y;
// 첫 번째 - 이차원 벡터 입력 방법
v.push_back({x, y});
// 두 번째 - 이차원 벡터 입력 방법
// v.push_back(make_pair(x, y));
}
sort(v.begin(), v.end(), compare); // 오름차순
for (int i = 0; i < n; i++) {
cout << v[i].first << " "<< v[i].second << "\n";
}
return 0;
}
세 번째 코드 - Array & 사용자 지정 Compare (O)
#include <iostream>
#include <algorithm>
using namespace std;
struct coordinate { // array 구조체 생성
int x, y;
};
bool compare(coordinate a, coordinate b) {
if (a.x == b.x) {
return a.y < b.y;
}
else {
return a.x < b.x;
}
}
struct coordinate arr[100001];
int main() {
int n, x, y;
cin >> n;
for (int i = 0; i < n; i++) {
cin >> arr[i].x >> arr[i].y;
}
sort(arr, arr+ n, compare);
for (int i = 0; i < n; i++) {
cout << arr[i].x << " " << arr[i].y << "\n";
}
return 0;
}
결과
11650번: 좌표 정렬하기
첫째 줄에 점의 개수 N (1 ≤ N ≤ 100,000)이 주어진다. 둘째 줄부터 N개의 줄에는 i번점의 위치 xi와 yi가 주어진다. (-100,000 ≤ xi, yi ≤ 100,000) 좌표는 항상 정수이고, 위치가 같은 두 점은 없다.
www.acmicpc.net
반응형
'Programming > C++ - 백준' 카테고리의 다른 글
[백준] 11656번 : 접미사 배열 | string.substr() 함수를 이용한 '부분 문자열 추출' (C++) (0) | 2024.03.14 |
---|---|
[백준] 10825번 : 국영수 (C++) (2) | 2024.03.14 |
[백준] 11931번 : 수 정렬하기 4 (C++) (0) | 2024.03.13 |
[백준] 11866번 : 요세푸스 문제 0 (C++) (0) | 2024.03.13 |
[백준] 10845번 : 큐 (C++) (0) | 2024.03.11 |
댓글